How to make Calculator Using HTML CSS and JavaScript
Simple Calculator Project Using HTML CSS and JavaScript
Firstly We can create a structure of calculator with the help of HTML
and Design and Now design our structure by using CSS or Add JavaScript Events for perform the task
successfully
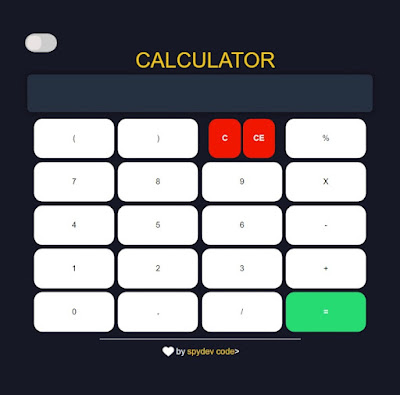 |
OUTPUT OF CALCULATOR |
Introduction
Briefly introduce the concept of creating a calculator using web technologies.
Explain that the tutorial will guide readers through the process step by step.
Highlight the importance of HTML, CSS, and JavaScript in web development.
Prerequisites
Mention what readers should already know (e.g., basic HTML, CSS, and JavaScript knowledge).
Provide links to relevant resources if readers need to brush up on these topics.
Step 1: Setting Up the HTML Structure
Create the HTML file and set up the basic structure.
Include the necessary HTML elements like <div>, <input>, and <button> for the calculator.
Step 2: Adding CSS Styles
Explain the importance of CSS in designing the calculator.
Create a separate CSS file and link it to the HTML.
Apply styles to the calculator elements for a visually appealing layout.
Step 3: Implementing the JavaScript Logic
Describe the role of JavaScript in making the calculator functional.
Create a JavaScript file and link it to the HTML document.
Begin by defining variables and functions.
Step 4: Handling User Input
Explain how to capture user input by clicking on buttons.
Use event listeners to detect button clicks.
Display the user's input on the calculator screen.
Step 5: Performing Calculations
Demonstrate how to implement basic arithmetic operations (addition, subtraction, multiplication, division) in JavaScript.
Provide code examples for each operation.
Update the screen with the results of calculations.
Step 6: Adding Clear and Equal Buttons
Include buttons to clear the screen and calculate results.
Explain how to handle these actions with JavaScript.
Step 7: Styling for Interactivity
Enhance the user experience by adding CSS styles for button hover and click effects.
Make the calculator visually responsive to user actions.
Step 8: Testing and Troubleshooting
Discuss the importance of testing the calculator's functionality.
Provide tips for debugging and solving common issues.
Conclusion
Summarize the key points of the tutorial.
Encourage readers to experiment with the calculator and make improvements.
Emphasize the importance of practice in web development.
Next Steps
Suggest additional features or functionalities that readers can add to their calculator.
Provide links to advanced tutorials or resources for further learning.
Final Thoughts
Express your excitement for readers who have successfully created their own calculator.
Encourage them to continue exploring web development and JavaScript.
Additional Tips:
Include code snippets with proper formatting and syntax highlighting.
Use clear and concise explanations.
Add images or diagrams to illustrate concepts.
Make the blog post SEO-friendly by including relevant keywords and meta tags.
This blog post will help your readers learn how to create a basic calculator using HTML, CSS, and JavaScript, providing them with valuable web development skills.
1 ) open Your Code Editor and Create New File index.html and paste the html code
It is important to create any project in html firstly you Need to
create HTML file With index.html you can customize the code Example
text color , background color , font Size , and you can add more function if You want .
copy code the whole HTML code and simply paste it
<!DOCTYPE html>
<html lang="en">
<head>
<meta charset="UTF-8" />
<meta
name="viewport"
content="width=device-width, height=device-height, initial-scale=1 user-scalable=no, shrink-to-fit=no"
/>
<meta http-equiv="X-UA-Compatible" content="ie=edge" />
<title>Simple Calculator</title>
<link rel="stylesheet" href="./styles/style.css" />
</head>
<body>
<div class="toggle-container">
<label class="toggle-switch">
<input type="checkbox" id="toggle-mode" />
<span class="toggle-slider"></span>
</label>
</div>
<div class="container">
<h1>
<a
style="
text-decoration: none;
color: #f1c40f;
margin-left: 1.25rem;
cursor: pointer;
"
onclick="location.reload();"
>CALCULATOR</a
>
</h1>
<div class="calculator">
<input type="text" name="screen" id="answer" readonly />
<table>
<tr>
<td><button>(</button></td>
<td><button>)</button></td>
<td>
<div class="row">
<div class="col">
<button
style="
background-color: #f01600;
font-weight: bold;
color: #ecf0f1;
width: 8vw;
"
onclick="clearAll()"
>
C
</button>
</div>
<div class="col">
<button
style="
background-color: #f01600;
font-weight: bold;
color: #ecf0f1;
width: 8vw;
"
onclick="deleteLastEntry()"
>
CE
</button>
</div>
</div>
</td>
<td><button>%</button></td>
</tr>
<tr>
<td><button>7</button></td>
<td><button>8</button></td>
<td><button>9</button></td>
<td><button>X</button></td>
</tr>
<tr>
<td><button>4</button></td>
<td><button>5</button></td>
<td><button>6</button></td>
<td><button>-</button></td>
</tr>
<tr>
<td><button>1</button></td>
<td><button>2</button></td>
<td><button>3</button></td>
<td><button>+</button></td>
</tr>
<tr>
<td><button>0</button></td>
<td><button style="font-weight: bold">.</button></td>
<td><button>/</button></td>
<td>
<button
style="
background-color: #25db72;
font-weight: bold;
color: #ecf0f1;
"
>
=
</button>
</td>
</tr>
</table>
<hr style="max-width: 50vw" />
<div
style="
font-size: 1rem;
display: flex;
align-items: center;
justify-content: center;
"
>
</div>
</div>
</div>
<div id="bar1" class="bars"></div>
<div id="bar2" class="bars"></div>
<div id="history"></div>
<div id="turn">PLEASE TURN YOUR DEVICE</div>
</body>
</script>
</html>
2) Now Create another file name style.css
in this file you can custmize your calculator
paste CSS Code Before adding CSS code Link Your CSS File With your HTML Page Kindly check Your HTML Page It is a Important for some times we forgot to link stylesheet page so
body,
html {
padding: 0;
margin: 0;
display: flex;
justify-content: center;
color: #ecf0f1;
background-color: rgb(23, 24, 37);
font-family: 'Fjalla One', sans-serif;
animation: fadein 1.5s;
font-family: 'Fjalla One', sans-serif;
}
@keyframes fadein {
0% {
opacity: 0%;
}
100% {
opacity: 100%;
}
}
h1 {
font-size: 2.5rem;
font-weight: 500;
margin-bottom: 0;
}
input {
background-color: rgba(52, 73, 94, 0.5);
color: #ecf0f1;
outline: none;
text-align: right;
border: none;
font-size: 3rem;
width: 80vw;
margin-bottom: 0.5rem;
border-radius: 0.5rem;
padding: 0.5rem 1.5rem;
-webkit-box-shadow: 0px 0px 10px 0px rgba(0,0,0,0.5);
-moz-box-shadow: 0px 0px 10px 0px rgba(0,0,0,0.5);
box-shadow: 0px 0px 10px 0px rgba(0,0,0,0.5);
}
.container {
margin: auto;
text-align: center;
position: absolute;
top: 50%;
left: 50%;
transform: translate(-50%, -50%);
}
.calculator {
padding: 0.25rem;
display: inline-block;
}
table {
margin: auto;
}
button {
border: none;
background-color: white;
width: 20vw;
height: 10vh;
padding: 0.5rem 0;
margin: 0.25vmax;
font-size: 2vmax;
border-radius: 1rem;
-moz-transition: all ease 0.5s;
-webkit-transition: all 0.5s ease;
transition: all 0.5s ease;
-o-transition: all ease 0.5s;
}
button:active {
transform: scale(0.95);
/* Scaling button to 0.98 to its original size */
box-shadow: 3px 2px 22px 1px rgba(0, 0, 0, 0.24);
/* Lowering the shadow */
}
button:hover {
-webkit-box-shadow: 0px 0px 10px 0px rgba(0,0,0,0.5);
-moz-box-shadow: 0px 0px 10px 0px rgba(0,0,0,0.5);
box-shadow: 0px 0px 10px 0px rgba(0,0,0,0.5);
-moz-transition: all ease 0.5s;
-webkit-transition: all 0.5s ease;
transition: all 0.5s ease;
-o-transition: all ease 0.5s;
}
a{
outline: none;
-moz-transition: all ease 0.5s;
-webkit-transition: all 0.5s ease;
transition: all 0.5s ease;
-o-transition: all ease 0.5s;
}
a:hover{
text-shadow: 0px 0px 10px rgba(241, 196, 15, 0.5);
-moz-transition: all ease 0.5s;
-webkit-transition: all 0.5s ease;
transition: all 0.5s ease;
-o-transition: all ease 0.5s;
}
#turn {
display: none;
z-index: 100;
position: fixed;
}
#historybutton{
width:40px;
height:40px;
border-radius: 20px;
float:right;
margin-right:15px;
cursor: pointer;
}
#history{
position: absolute;
width:90%;
height: 80vh;
top:10vh;
background-color: white;
border: 6px solid black;
border-radius: 10px;
display: none;
/* overflow-y: scroll; */
}
.historyelement{
color: black;
margin: 20px;
font-size: 40px;
}
#bar1, #bar2{
position: absolute;
width:30px;
height: 4px;
background-color: white;
margin-top: 20px;
margin-right: 20px;
margin-left: 93%;
transform: rotate(45deg);
cursor: pointer;
display: none;
}
#bar2{
transform: rotate(135deg);
}
@media (orientation: landscape) and (max-height: 500px) {
#turn {
width: 100vw;
height: 100vh;
display: flex;
justify-content: center;
align-items: center;
font-size: 2rem;
color: rgba(52, 73, 94, 1.0);
background-color: rgba(236, 240, 241, 1.0);
}
}
.toggle-container {
position: absolute;
top: 4rem;
left: 3rem;
}
.toggle-switch {
position: relative;
display: inline-block;
width: 60px;
height: 34px;
}
.toggle-switch input {
opacity: 0;
width: 0;
height: 0;
}
.toggle-slider {
position: absolute;
cursor: pointer;
top: 0;
left: 0;
right: 0;
bottom: 0;
background-color: #ccc;
-webkit-transition: .4s;
transition: .4s;
border-radius: 34px;
}
.toggle-slider:before {
position: absolute;
content: "";
height: 26px;
width: 26px;
left: 4px;
bottom: 4px;
background-color: rgb(233, 227, 227);
-webkit-transition: .4s;
transition: .4s;
border-radius: 50%;
border-radius: 10px;
}
input:checked + .toggle-slider {
background-color: #2a2c2e;
border-radius: 10px;
}
input:focus + .toggle-slider {
box-shadow: 0 0 1px #2b2c2d;
border-radius: 10px;
}
input:checked + .toggle-slider:before {
-webkit-transform: translateX(26px);
-ms-transform: translateX(26px);
transform: translateX(26px);
}
/* Dark mode styles */
.dark-mode {
background-color: black;
border-radius: 10px;
color: #fff;
}
.dark-mode body,
.dark-mode html {
background-color: black;
border-radius: 10px;
}
.dark-mode .calculator {
background-color: black;
border-radius: 10px;
}
.dark-mode table {
color: #fff;
border-radius: 10px;
}
.dark-mode button {
background-color: #555;
color: #fff;
border-radius: 10px;
}
.dark-mode a {
color: #fff;
border-radius: 10px;
}
.row{
display: flex;
font-size: 1rem;
justify-content: center;
max-width: 20vw;
margin: auto;
}
#answer {
caret-color: #0f38f1;
}
/* Negative Numbers */
.negative {
color: red;
}
2) Here Now Create Your JavaScript Page With name script.js name , Now link Your JavaScript File With HTML File Inside Body tag add your JavaScript file on Load your browser Successfully.
window.dataLayer = window.dataLayer || [];
function gtag() {
dataLayer.push(arguments);
}
gtag("js", new Date());
gtag("config", "UA-70447982-5");
</script>
<script>
const toggleMode = document.getElementById("toggle-mode");
const container = document.querySelector(".container");
toggleMode.addEventListener("change", function () {
container.classList.toggle("dark-mode");
});
document.addEventListener("keydown", function (event) {
handleKeyPress(event.key);
});
// This function will be responsible for handling the button press from the keyboard..Try thses key also if want you can also chnage these settings,...
function handleKeyPress(key) {
// "Enter" key is pressed, trigger the "=" button press
if (key === "Enter") {
handleButtonPress("=");
}
// "Delete" or "Backspace" key is pressed, trigger the "CE" button press
if (key === "Delete" || key === "Backspace") {
handleButtonPress("CE");
}
//number key is pressed, trigger the corresponding number button press
if (/[0-9]/.test(key)) {
handleButtonPress(key);
}
// operator key is pressed (+, -, *, /), trigger the corresponding operator button press
if (/[\+\-\*\/%]/.test(key)) {
handleButtonPress(key);
}
}
function handleButtonPress(value) {
// This function simulates the button press of the calculator for the given value
// Find the corresponding button element based on the value
const button = document.querySelector(`button[value="${value}"]`);
if (button) {
button.click();
}
}
tags : web development projects with source code , web development projects , CSS Animations , JavaScript Projects With source code.
Related Post :
10 Mind-Blowing CSS Animation Examples (Free Code + Demos).
JavaScript Programming Syntax Tips.
Create a Stunning 3D Carousel with HTML, CSS, and JavaScript.
Comments
Post a Comment
If you have any Suggestions For Me .Plese let me know